Open digital diary
Real events and Imagination that are open to all
googleAd
Python for Beginners | Chapter 8 | Conditions Explained
The Latest Technology Trends: Virtual and Augmented Reality, Artificial Intelligence, 5G and IoT, Blockchain
Course Outline for Python Beginner course
Before learning a course, knowing the course outline will prepare you with high level concepts that you are going to learn. In this post, you are going to see the key chapter outlines you will find in many Python beginner courses. These may not be exact chapter names and also some courses may have some additions or omissions on these. But this should give you fairly a good idea on what you'll be learning.
Chapter 1: Introduction to Python
- What is Python?
- Why use Python?
- How to install and set up Python
- Basic syntax and data types
Chapter 2: Variables and Data Types
- Understanding variables
- Basic data types (numbers, strings, lists, etc.)
- Typecasting
- Basic math operations
Chapter 3: Control Flow
- If-else statements
- For and while loops
- Break and continue statements
Chapter 4: Functions
- Defining and calling functions
- Parameters and arguments
- Return statement
- Scope of variables
Chapter 5: Modules and Packages
- Importing modules
- Creating and using packages
- Standard library modules
Chapter 6: File I/O
- Opening and reading files
- Writing to files
- Working with CSV and JSON files
Chapter 7: Exception Handling
- Understanding exceptions
- Try-except-else-finally block
- Raising exceptions
Chapter 8: Object-Oriented Programming
- Classes and objects
- Constructors and destructors
- Inheritance and polymorphism
Chapter 9: Advanced Topics
- Decorators
- Generators
- Lambda functions
- List comprehensions
Chapter 10: Project Development
- Planning and organizing a project
- Debugging and testing
- Deployment and distribution
Note: The above course is a brief introduction to Python language and its features. The chapters are designed to give an overview of the concepts and not a deep dive. The actual course would be much more detailed and would include examples and exercises for each chapter, and it would be run for a longer time for the students to practice and master the concepts.
Python for Beginners - Learn Python Without IDE - 2 (Variables)
Python beginner tutorial encourages you to learn by doing. Follow along this Python course to get started. This is Part 2 of the python full course for beginners which just gets you started by showing how to install python on your machine and write your very first program. Subsequent parts will follow shortly.
I have chosen not to use any python specific IDE (Integrated Development Environment) in this course. IDEs tend to do lots of autocomplete which hinders learning. As you get familiar with python basics, programming concepts and get comfortable writing python programs without an IDE, you will find IDEs more productive later. As a python beginner, start from the scratch. I value all feedbacks. If you liked this method of teaching or have any constructive criticism, please share that in the comments. Please subscribe to be notified of the next part of the course.Python for Beginners - Learn Python Now Without IDE
Python Tutorial for beginners encourages you to learn by doing. Follow along this Python course to get started. This is Part 1 of the python full course for beginners which just gets you started by showing how to install python on your machine and write your very first program. Subsequent parts will follow shortly. I have chosen not to use any python specific IDE (Integrated Development Environment) in this course. IDEs tend to do lots of autocomplete which hinders learning. As you get familiar with python basics, programming concepts and get comfortable writing python programs without an IDE, you will find IDEs more productive later. As a python beginner, start from the scratch. I value all feedbacks. If you liked this method of teaching or have any constructive criticism, please share that in the comments. Please subscribe to be notified of the next part of the course.
Below is my free course on python. Enjoy!
Installing Raspberry Pi from Canakit
Raspberry Pi is a wonderful mini computer that consumes less power and can be a very powerful machine for a variety of projects like running your own vpn server or running a web server, media server or game server. Raspberry Pi has become more and more powerful over the years. It is extremely tiny making it very portable. We will see how to setup a Raspberry Pi device. I bought mine from Canakit.
After burning my first Pi after heavily loading it with accessories, I decided to be a bit careful on my 2nd device. On my first Pi, I had only bought the board and I used my own power cord. This time I bought all the necessary accessaries from Canakit, including pre-installed Raspian OS, a case, heat sink, a fan and a power cord.
Mine is a 8 GB Ram and I got a 128 GB sd card which comes with pre-installed Raspian OS. It was very easy to install the Raspberry pi board on to the case. Also installing the fans, heat sinks and connecting with the power cord was super easy.
Below is a video demonstrating the setup.
Kansas City Zoo - Review
A few days back we had gone to the Kansas City Zoo, a trip that was very worthy of our time and money.
My 4 year old enjoyed identifying all the animals she had seen on her books and Tv. This zoo is bigger than the Topeka zoo and even the St. Louis zoo. It is 202 acres in size and houses around 1700 animals; so you can plan your entire day for this zoo.
I was really surprised to see some Polar Bear, Jelly Fish and Penguins in this zoo. However Polar bear wouldn't be bleach-white you would have seen on movies. I had thought Polar bears can live only in the Arctic and the Penguins can live only in the Antarctic. Kansas City Zoo proved it can live anywhere with the right temperature settings.
The Penguins were in a temperature controlled glass rooms which had both snow in one section and a large swimming area in the other.
I hadn't seen a Jelly fish so up close before, so it was a visual treat.
The Sea Lions demonstrated some acrobatics while the their coach narrated their life story. See video below
The zoo is divided into sections where it houses animals from different geographical locations. Australia and Africa the popular ones. You can either walk across the zoo or use one of their trams. We bought a platinum pass that gave access to their African Tram, African Sky Safari, Carousel of Endangered Species, KC Zoo Railroad and the Kenyan Cruise.
African Sky Safari:
View of the Zebras from Sky Safari
Feeding the Goats in Kansas City Zoo
You can buy tickets online for both admission and the rides on kansas city zoo website - https://www.kansascityzoo.org/
Python for Beginners | Chapter 8 | Conditions Explained
In this chapter we will learn how condition statements work in python. If you are new in here, you may want to start from the beginning...
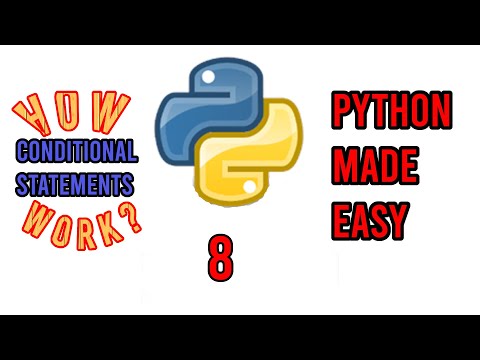
-
நீங்கள் வேறு ஒரு மாநிலத்திற்கோ அல்லது நாட்டிற்கோ செல்லும் போது, புதுப்புது அனுபவங்கள் கிடைத்திருக்கும். சில விஷயங்கள் வித்தியாச...
-
I am here in Kansas to attend a few business meetings in Topeka. Seeing the Kansas city International airport after a long time. Reminded of...
-
In this chapter we will learn how condition statements work in python. If you are new in here, you may want to start from the beginning...